Texture code
Your repository will contain a 'Textures' template code. Fill in all the TODO blocks and map the Rose-Hulman image to the model. You will need to:
- Set the texture coordinates for the model
- Specify and upload the texture image data
- Activate and use a texture unit when rendering
- Make the shader use the texture coordinates and data
Hints
- You can adjust the view with the mouse.
- A wireframe outline of the model is also rendered. This can help you visualize the triangle boundaries.
- You can have the shader display the texture coordinates to make sure the are what you intend.
- Use these CPU side setup functions: glGenTextures, glBindTexture, glTexParameterf, glTexImage2D, glGenerateMipmap.
- Use these CPU side render functions: glActiveTexture.
- Use these GPU side render functions: texture.
Code
The code from in class:RenderEngine.h : display()
//TODO bind the texture object glBindTexture(GL_TEXTURE_2D, textures[i]); //TODO activate a texture unit GLint texUnitId = 0; glActiveTexture(GL_TEXTURE0+texUnitId); //TODO bind the sampler to a texture unit glUniform1i(glGetUniformLocation(shaderProg[0], "texUnit"), texUnitId); glDrawArrays(GL_TRIANGLES, 6*i, 6); glBindTexture(GL_TEXTURE_2D, 0);
RenderEngine.h : setupTextures()
//TODO bind a texture object glBindTexture(GL_TEXTURE_2D, textures[i]); //TODO set min/mag sampling parameters glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_NEAREST); //glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR); //glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR_MIPMAP_LINEAR); glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_NEAREST); //glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR); //TODO set edge wrap parameters glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE); glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE); //glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_MIRRORED_REPEAT); //glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_MIRRORED_REPEAT); //glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT); //glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT); //TODO upload texture and generate mipmap glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA, texSizeX, texSizeY, 0, GL_RGBA, GL_UNSIGNED_BYTE, texData); bool mipmapEnabled = true; if(mipmapEnabled) { //mip mapping, upload 0 level, then build maps glGenerateMipmap(GL_TEXTURE_2D); } else { //no mip mapping, upload 0 level only } //TODO unbind the texture glBindTexture(GL_TEXTURE_2D, 0);
simple.frag
//TODO upload a uniform sampler2D texture sampler id uniform sampler2D texSampler; ... //TODO use the texture() function with uploaded sampler id to get a texture color fragColor = texture(texSampler, texMapping);
Rubric
Texture rectangle with Rose-Hulman image | 0: No texturing | 5: Texture uploaded and used, but coords incorrect | 10: Correct image displayed |
Example
This image is everything working with the default texture coordinates: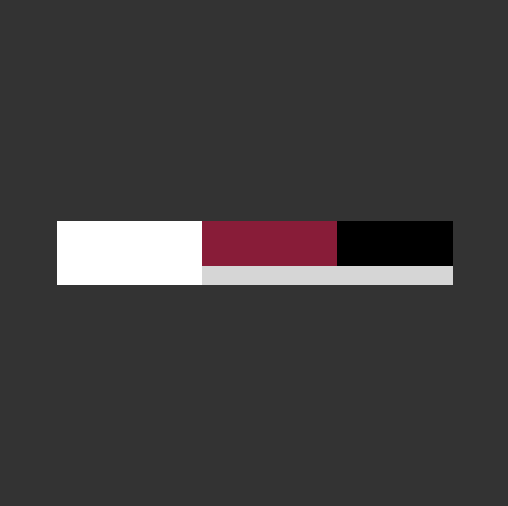
This image is everything working:
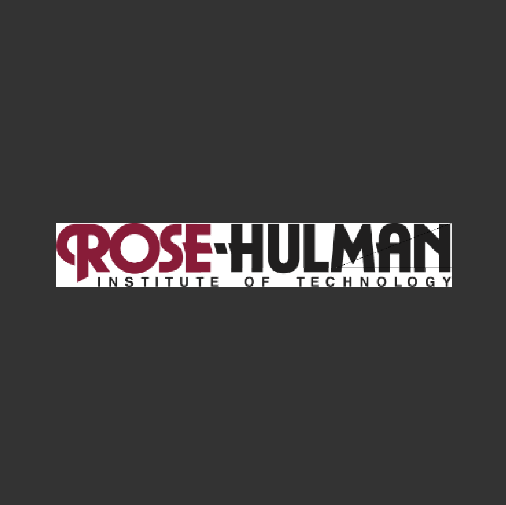